I’m trying to access struct declarations:
const Type = struct {
const decl1 = void;
const decl2 = void;
const decl3 = void;
};
pub fn printOutDecls(comptime T: type) void {
std.log.debug("{any}", .{@typeInfo(T).Struct.decls});
}
pub fn main() !void {
printOutDecls(Type);
}
But unfortunately, I always get an empty list:
debug: { }
Any ideas why is that?
Just in case, according to docs, decls
is a slice of Declarations ([]const Declaration
), where Declaration is a struct with a name literal (const Declaration = { name: []const u8 }
).
Those aren’t declarations, those are privately namespaced type alias definitions. Not sure if there’s an easy way to print them out, though.
These would be public declarations:
const std = @import("std");
const Type = struct {
pub fn decl1() void {}
pub fn decl2() void {}
pub fn decl3() void {}
};
fn printOutDecls(comptime T: type) void {
for (@typeInfo(T).Struct.decls) |decl| {
std.debug.print("{s}\n", .{decl.name});
}
}
pub fn main() void {
printOutDecls(Type);
}
Em… I’m a bit confused 
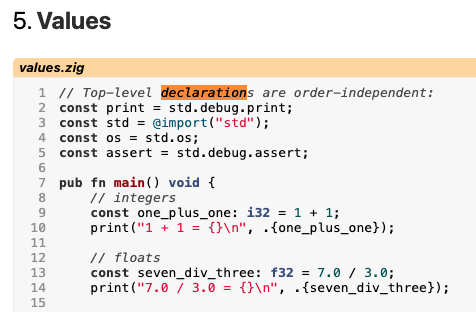
There isn’t much about what declaration really is but from the example above I thought that anything other than a field becomes a “declaration”. Seems I was making a wrong assumption.
Anyway, can I have access to those “privately namespaced type alias definitions” through the type information?
Oh, yeah, my bad 
Here, just make em public:
const std = @import("std");
const Type = struct {
pub const decl1 = void;
pub const decl2 = void;
pub const decl3 = void;
};
fn printOutDecls(comptime T: type) void {
for (@typeInfo(T).Struct.decls) |decl| {
std.debug.print("{s}\n", .{decl.name});
}
}
pub fn main() void {
printOutDecls(Type);
}
1 Like